Overview
Incident Management System is a desktop application used by emergency call operators for managing incidents. The user interacts with it via CLI. It is written in Java, has a JavaFX GUI, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Implemented Incident Filling and Submission
-
What it does: Allows users to identify and subsequently fill and/or submit incident reports in the system.
-
Justification: This enhancement improves the product by (1) fulfilling its most basic function of reporting incidents, (2) categorising incidents into 'drafts' which can be filled before they become 'submitted reports' officially registered in the system, and (3) providing users a convenient way to list incidents ready for filling and submission.
-
Highlights: This enhancement affects existing commands and modifies the Incident model (and hence, UI) behaviour. It is designed so it can be easily extended to accommodate changes to the Incident model. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required brainstorming a seamless way of tracking changes to the status of incidents.
-
-
Minor enhancement: Implemented Incident and Vehicle GUI layout
-
Summary: allows users to view incident reports and on-duty vehicles side-by-side in a readable format.
-
Highlights: this enhancement required in-depth understanding of the GUI design and JavaFX syntax.
-
-
Code contributed: [View on RepoSense]
-
Other contributions:
-
Project management: Integrated Travis CI to the team repo
-
Team task: Created initial UI mockup of the product [#16]
-
Contributions to the User Guide
_Given below is one of the sections I contributed to the User Guide. It showcases my ability to write documentation targeting end-users. |
Filling a draft incident report: fill
Fills a draft incident report if relevant parameters are specified, otherwise lists all draft reports ready for filling.
This command works in two modes:
-
Outcome of
fill
:
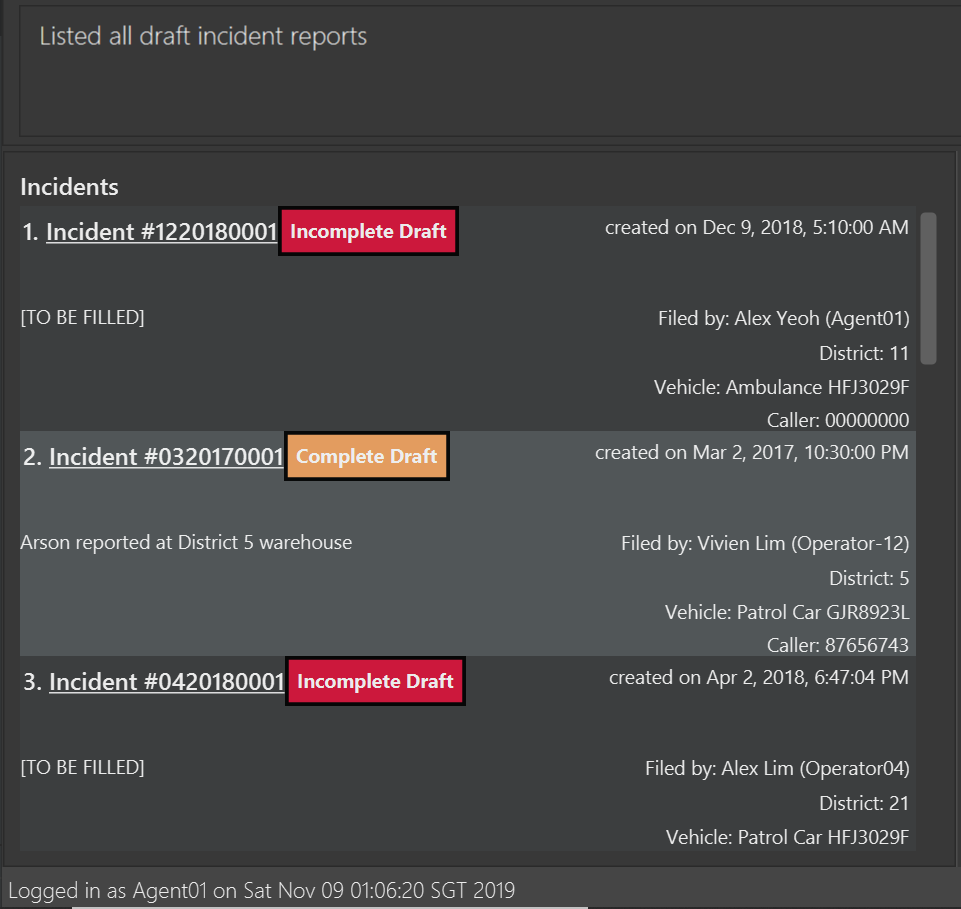
-
Outcome of
fill 1 p/90309049 desc/Traffic accident reported at FKU hospital junction
:
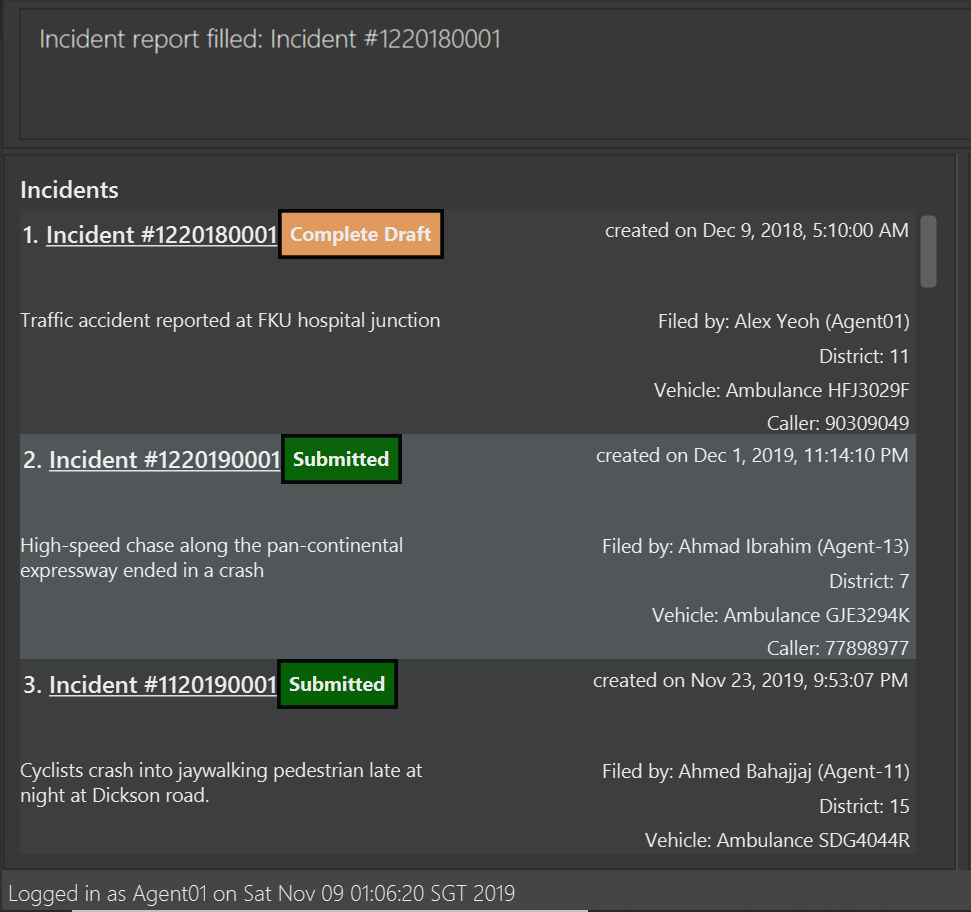
Using fill
in both modes one after the other thus enables convenient listing and filling of target incident reports.
Tracking incident history [coming in v2.0]
I also wrote section 3.12 of the User Guide explaining the functioning of the submit command in two modes.
|
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Incident Filling and Submission feature
Implementation
The incident filling and submission subroutines are facilitated by the fill
and submit
commands respectively.
In the IMS, each incident can have one of three statuses - INCOMPLETE_DRAFT
, COMPLETE_DRAFT
, and SUBMITTED REPORT
.
These three statuses are maintained by an enum
in Incident
. Executing the fill
command changes the status of
drafts (complete or incomplete) into COMPLETE_DRAFT
while the submit
command changes the status of only COMPLETE_DRAFTS
to SUBMITTED_REPORT
.
To prevent potential misuse, only the operator who has created the incident report is allowed access to execute the fill and submit commands on that report.
Overview of Fill and Submit commands
Each command works in two modes:
-
Without parameters: In this mode, the command -
fill
orsubmit
- lists the incidents that are ready for Filling (i.e. only all complete and incomplete drafts) or Submitting (i.e. only all complete drafts). -
With parameters: In this mode, the command -
fill
orsubmit
- actually fills (i.e. makes incident statusCOMPLETE_DRAFT
) or submits (i.e. changes incident status fromCOMPLETE_DRAFT
toSUBMITTED_REPORT
) the specified incident.
The implementation of these two modes is discussed below. As both fill
and submit
are rather similar in their implementation, a detailed discussion of only the fill
command is given below.
No parameter mode (listing incidents)
This mode leverages the ability of the ListIncidentsCommand
to list incidents by different predicates.
When the IncidentManagerParser
parses a fill
command without parameters, it returns a new ListIncidentsCommand
with predicate Incident::isDraft
. This ListIncidentsCommand
is then executed as per usual.
For the submit
command, the predicate Incident::isCompleteDraft
is used instead.
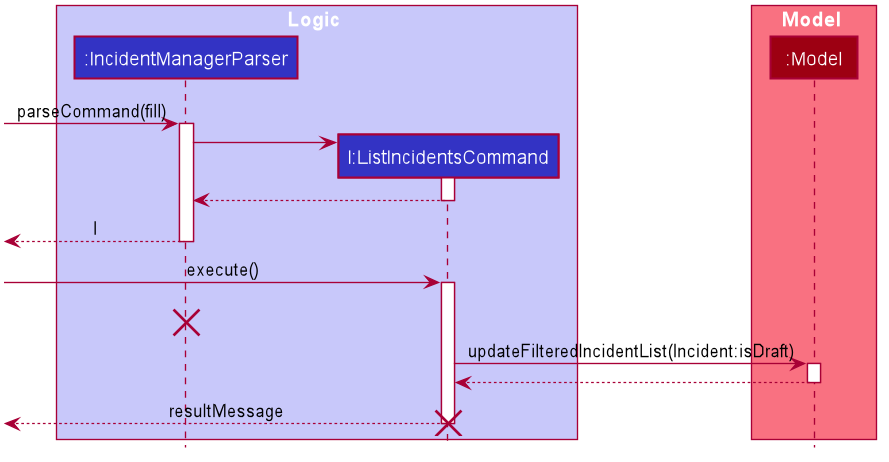
Parameter mode (modifying incidents)
-
For the
fill
command with parameters, theFillCommandParser
will be invoked to parse the fieldstargetIndex
,callerNumber
, anddescription
and return aFillCommand
containing these non-null fields. -
The
execute()
method inFillCommand
will then retrieve the specified incident if there are drafts to be filled and if the index is valid. -
Two helper methods -
processReportFilling
andfillReport
- will complete the filling process.fillReport
returns a newIncident
which is a copy of the incident report to be filled, but with the specified caller and description details and aCOMPLETE_DRAFT
status. -
The old incident report will be removed from the system and be replaced with the new updated incident report.
-
The new incident report is placed at the front of the incident list for easy access.
In this sequence diagram, the helper methods within FillCommand
are omitted for clarity.
The SubmitCommand
functions similarly, with one crucial difference. As no Incident fields are to be updated, the specified incident is simply retrieved, and its fields are copied into a new Incident
object with a SUBMITTED_REPORT
status.
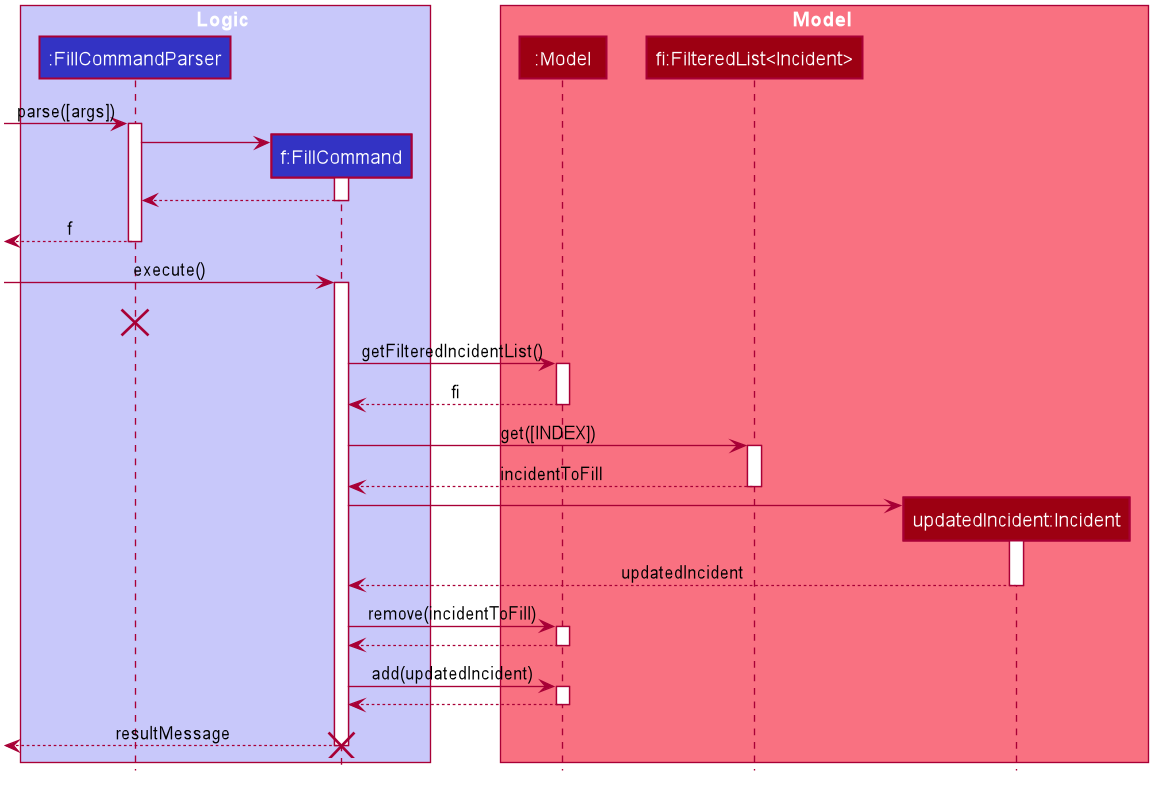
Design Considerations
Aspect: How incident Fill and Submit commands execute in no parameter mode
-
Current choice: Use
ListIncidents
command with appropriate predicate to fulfillfill
andsubmit
functionalities in no-parameter mode.-
Pros:
-
Intuitive and convenient to use. If user needs easy access to reports that can be filled or submitted, they do not need to remember a new command keyword.
-
Requires lesser code. Abstraction of the filtered listing subroutine reduces the amount of redundant code.
-
-
Con: 1. Might be potentially confusing to user as
FillCommand
is performing a function of listing that is extraneous to the function of filling.
-
-
Alternative 1: Extend
FillCommand
to create two child classesFillCommandNoParams
andFillCommandWithParams
.-
Pros:
-
Better use of the OOP principle of inheritance.
-
Reduce coupling between
ListIncidentsCommand
andFillCommand
.
-
-
Cons:
-
Increases amount of code and hence marginally reduces app performance as one additional new class needs to be created.
-
Misleading use of abstraction as the
FillCommandNoParams
is technically not performing the function of filling but that of listing.
-
-
-
Alternative 2: Separate the 'listing' and the 'filling' aspect by using separate command words.
-
Pro: 1. Most appropriate use of abstraction and single responsibility principle, which are crucial OOP concepts.
-
Con: 1. User needs to either remember an additional command word or type a longer
list-i
command by specifying the filter predicate, which reduces user convenience.
-
Aspect: How incident Fill and Submit commands execute in parameter mode
-
Current choice: Both
callerNumber
anddescription
fields need to be specified when filling specified incident report. The other incident report fields are auto-filled and can only be changed by using theedit
command once the incident report has been submitted.-
Pros:
-
Improved accountability. Prevents a user from changing the most important fields of the incident report, such as
incidentId
,incidentDateTime
, andvehicle
, without first committing the report into the system. -
More convenient for the user as they only have to specify 2 report fields instead of 6 or 7.
-
-
Con: 1. User is unable to fill
callerNumber
independently ofdescription
unless they first submit the incident report and then use theedit
command.
-
-
Alternative 1: Combine
fill
andsubmit
functions i.e. filling a report completely will automatically submit it.-
Pros:
-
Easier to implement as Incident reports have two statuses - DRAFT or SUBMITTED - instead of three.
-
More convenient as this results in one less step in the user’s workflow and one less command word for the user to remember.
-
-
Con: 1. Less adaptable and modular. If new fields are added to the incident report, then the user might want to enter / replace those fields by executing repeated fill commands without committing the report into the system with incomplete / likely to change information.
-
-
Alternative 2: Allow
fill
command to fill variable number of fields.-
Pro: 1. Satisfies the cons of the two approaches above as it is versatile enough to allow the user to independently fill different incident report fields as well as adaptable enough to accommodate extra fields.
-
Con: 1 . Harder to implement as we would need more elaborate methods to parse the variable arguments.
-
Known Issues
A user cannot independently fill the various incident report fields unless they first submit the incident report. This might be an acceptable issue it encourages users to completely fill a new incident report before submitting it, which reduces the likelihood of finding incomplete drafts in the system.
Activity diagram summarising Incident creation, filling, and submission features
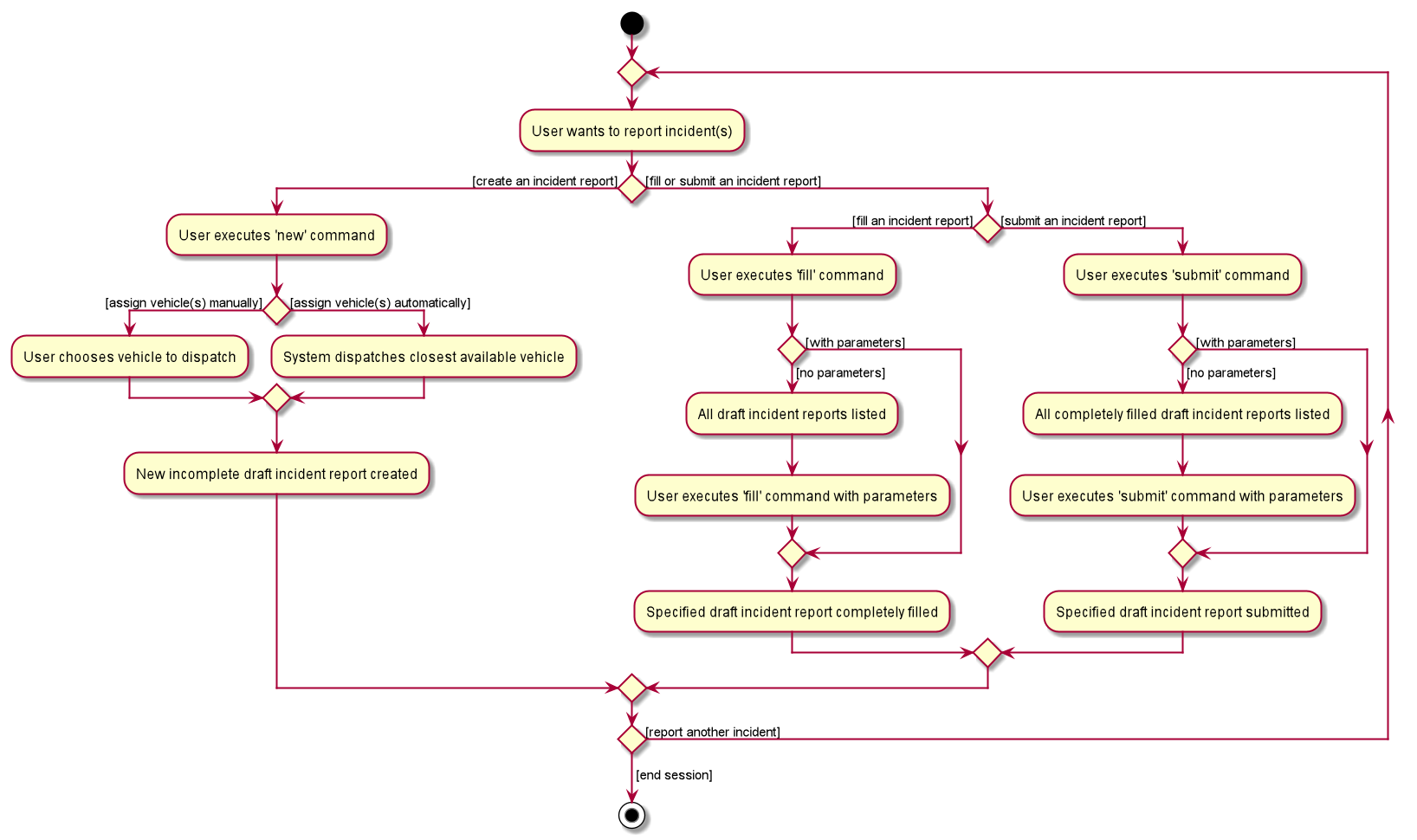
In this activity diagram, the catch-all term 'report' is used to encompass the acts of creating, filling, and submitting incident reports.
Incident component
The Incident
:
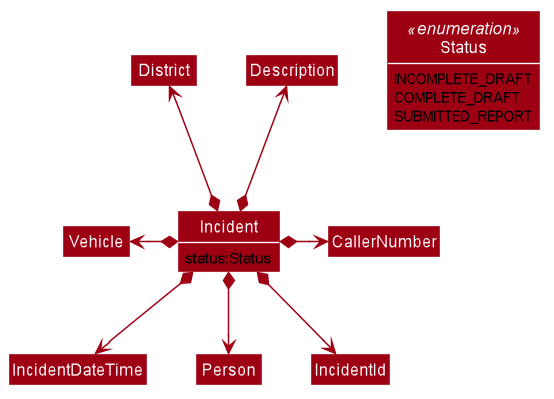
-
Represents an incident report in the incident manager.
-
Contains the attributes
CallerNumber
,Description
,IncidentDateTime
and IncidentId`. -
Also contains a
Person
object representing the 'Operator' who filed the incident, aDistrict
which represents the location of the incident, and aVehicle
representing the vehicle dispatched to investigate this incident. -
Has three states encapsulated by a
Status
enum -INCOMPLETE_DRAFT
(report not completely filled and not submitted),COMPLETE_DRAFT
(report completely filled but not submitted), andSUBMITTED_REPORT
(report completely filled and submitted).